pep8 is a tool to check your Python code against some of the styleconventions in PEP 8.
This document gives coding conventions for the Python code comprising the standard library in the main Python distribution. Please see the companion informational PEP describing style guidelines for the C code in the C implementation of Python. This document and PEP 257 (Docstring Conventions) were adapted from Guido's original Python Style Guide essay, with some additions from. PEP 8 is Python's style guide. It's a set of rules for how to format your Python code to maximize its readability. Writing code to a specification helps to make large code bases, with lots of writers, more uniform and predictable, too. Pep 8 をみんなが守っていて「文法」に準じるくらいの権威を持っている 気がします。 pep 8 という言葉そのものは覚えておいても 損はないかもなという感覚がなんとなく伝わっていれば幸いです。 ここまでお付き合いいただき、誠にありがとうございました。. Code like Guido? Free Live Syntax Checker (Python PEP8 Standard). Pep8 is a tool to check your Python code against some of the style conventions in PEP 8.
- Plugin architecture: Adding new checks is easy.
- Parseable output: Jump to error location in your editor.
- Small: Just one Python file, requires only stdlib. You can use justthe pep8.py file for this purpose.
- Comes with a comprehensive test suite.
This utility does not enforce every single rule of PEP 8. It helps toverify that some coding conventions are applied but it does not intendto be exhaustive. Some rules cannot be expressed with a simple algorithm,and other rules are only guidelines which you could circumvent when youneed to.
Always remember this statement from PEP 8:
Among other things, these features are currently not in the scope ofthe pep8
library:
- naming conventions: this kind of feature is supported through plugins.Install flake8 and thepep8-naming extension to usethis feature.
- docstring conventions: they are not in the scope of this library;see the pep257 project.
- automatic fixing: see the section PEP8 Fixers in therelated tools page.
You can install, upgrade, uninstall pep8.py with these commands:
There's also a package for Debian/Ubuntu, but it's not always thelatest version:
You can also make pep8.py show the source code for each error, andeven the relevant text from PEP 8:
Or you can display how often each error was found:
You can also make pep8.py show the error text in different formats by using –format having options default/pylint/custom:
Variables in the custom
format option
Variable | Significance |
---|---|
path | File name |
row | Row number |
col | Column number |
code | Error code |
text | Error text |
Quick help is available on the command line:
The behaviour may be configured at two levels, the user and project levels.
At the user level, settings are read from the following locations:
- If on Windows:
~.pep8
- Otherwise, if the
XDG_CONFIG_HOME
environment variable is defined: XDG_CONFIG_HOME/pep8
- Else if
XDG_CONFIG_HOME
is not defined: ~/.config/pep8
Example:
At the project level, a setup.cfg
file or a tox.ini
file is read ifpresent (.pep8
file is also supported, but it is deprecated). If none ofthese files have a [pep8]
section, no project specific configuration isloaded.
This is the current list of error and warning codes:
code | sample message |
---|---|
E1 | Indentation |
E101 | indentation contains mixed spaces and tabs |
E111 | indentation is not a multiple of four |
E112 | expected an indented block |
E113 | unexpected indentation |
E114 | indentation is not a multiple of four (comment) |
E115 | expected an indented block (comment) |
E116 | unexpected indentation (comment) |
E121 (*^) | continuation line under-indented for hanging indent |
E122 (^) | continuation line missing indentation or outdented |
E123 (*) | closing bracket does not match indentation of opening bracket's line |
E124 (^) | closing bracket does not match visual indentation |
E125 (^) | continuation line with same indent as next logical line |
E126 (*^) | continuation line over-indented for hanging indent |
E127 (^) | continuation line over-indented for visual indent |
E128 (^) | continuation line under-indented for visual indent |
E129 (^) | visually indented line with same indent as next logical line |
E131 (^) | continuation line unaligned for hanging indent |
E133 (*) | closing bracket is missing indentation |
E2 | Whitespace |
E201 | whitespace after ‘(‘ |
E202 | whitespace before ‘)' |
E203 | whitespace before ‘:' |
E211 | whitespace before ‘(‘ |
E221 | multiple spaces before operator |
E222 | multiple spaces after operator |
E223 | tab before operator |
E224 | tab after operator |
E225 | missing whitespace around operator |
E226 (*) | missing whitespace around arithmetic operator |
E227 | missing whitespace around bitwise or shift operator |
E228 | missing whitespace around modulo operator |
E231 | missing whitespace after ‘,', ‘;', or ‘:' |
E241 (*) | multiple spaces after ‘,' |
E242 (*) | tab after ‘,' |
E251 | unexpected spaces around keyword / parameter equals |
E261 | at least two spaces before inline comment |
E262 | inline comment should start with ‘# ‘ |
E265 | block comment should start with ‘# ‘ |
E266 | too many leading ‘#' for block comment |
E271 | multiple spaces after keyword |
E272 | multiple spaces before keyword |
E273 | tab after keyword |
E274 | tab before keyword |
E3 | Blank line |
E301 | expected 1 blank line, found 0 |
E302 | expected 2 blank lines, found 0 |
E303 | too many blank lines (3) |
E304 | blank lines found after function decorator |
E4 | Import |
E401 | multiple imports on one line |
E402 | module level import not at top of file |
E5 | Line length |
E501 (^) | line too long (82 > 79 characters) |
E502 | the backslash is redundant between brackets |
E7 | Statement |
E701 | multiple statements on one line (colon) |
E702 | multiple statements on one line (semicolon) |
E703 | statement ends with a semicolon |
E704 (*) | multiple statements on one line (def) |
E711 (^) | comparison to None should be ‘if cond is None:' |
E712 (^) | comparison to True should be ‘if cond is True:' or ‘if cond:' |
E713 | test for membership should be ‘not in' |
E714 | test for object identity should be ‘is not' |
E721 (^) | do not compare types, use ‘isinstance()' |
E731 | do not assign a lambda expression, use a def |
E9 | Runtime |
E901 | SyntaxError or IndentationError |
E902 | IOError |
W1 | Indentation warning |
W191 | indentation contains tabs |
W2 | Whitespace warning |
W291 | trailing whitespace |
W292 | no newline at end of file |
W293 | blank line contains whitespace |
W3 | Blank line warning |
W391 | blank line at end of file |
W5 | Line break warning |
W503 | line break occurred before a binary operator |
W6 | Deprecation warning |
W601 | .has_key() is deprecated, use ‘in' |
W602 | deprecated form of raising exception |
W603 | ‘<>' is deprecated, use ‘!=' |
W604 | backticks are deprecated, use ‘repr()' |
(*) In the default configuration, the checks E121, E123, E126,E133, E226, E241, E242 and E704 are ignored because theyare not rules unanimously accepted, and PEP 8 does not enforce them. Thecheck E133 is mutually exclusive with check E123. Use switch --hang-closing
to report E133 instead of E123.
(^) These checks can be disabled at the line level using the #noqa
special comment. This possibility should be reserved for special cases.
Note: most errors can be listed with such one-liner:
The flake8 checker is a wrapper aroundpep8
and similar tools. It supports plugins.
Other tools which use pep8
are referenced in the Wiki: list of relatedtools.
CS106A does not just teach coding. It has always taught how to write clean code with good style.Your section leader will talk with you about the correctness of code, but also pointers for good style.
Messy code works poorly — hard to read, hard to debug. To help you develop the right habits, we want you to always turn in clean code, and of course all of our examples should also have good clean style.
The goal of good style is 'readable' code, meaning that when someone sweeps their eye over the code, what that code does shines through.
Experience has shown that readable code is less time consuming to build and debug. Debugging especially can be a huge time-sink, so learning to write clean code and keep it clean is how we will do things all the time. Like surgeons keeping their hands clean, it's the right way to do it and we will try to develop the habit all the time.
Readability 1 - Function and Variable Names
The first step to good readability is a good variable and function names, like this example:
The narrative steps the code takes are apparent from the good function and variable names (essentially the verbs and nouns in the narrative). No other comments are necessary here. The names alone make it very readable.
The functions are the actions in the code, so we prefer verb names like 'display_alert' or 'remove_url' that say what the function does. Variables are like the nouns of the code, so we prefer names that reflect what that variable stores like 'urls' or 'original_image' or 'resized_image'. We'll talk about naming in more detail in the Style 2 document.
Readability 2 - PEP8 Tactics
Python is developed as a collaborative, free and open source project. A 'PEP' (Python Enhancement Proposal) is a written proposal used in Python development.
One of the earliest PEPs, PEP8, is a consensus set of style and formatting rules for writing 'standard' style Python, so your code has the right look for anyone else reading or modifying it. This is very much like English spelling reform, where 'public' is the standard spelling, and 'publick' is out of use and looks weird.
Here are the most important PEP8 rules we will use. The code you turn in should abide by PEP8.
Indent 4 Spaces
Indent code logically using spaces — 4 spaces is the most common.For if/for/while .. write 'body' lines indented on the next line after the colon.Early in the life of Python, some programs used 2 spaces and some used 4 spaces, but 4 spaces has grown to dominate. The best practice is to use literal spaces to indent the code, not tab stops.
Single Space Between Items
Most lines of code have a mix of variables and 'operators' such as as + * / = >=
. Use a single space to separate the operators and items:
Space After Comma/Colon
A comma or colon has 1 space after it, but no spaces before it.
Parenthesis No Space
The left and right parenthesis are not separated from what they contain, and the parenthesis for a function call is not separated from the name of the function. Similarly, square brackets and curly braces are not separated from what they contain.
Use a Consistent Quote Mark
Python strings can be written within single quotes like 'Hello'
or double quotes like'there'
. PEP8 requires a program to pick one quote style and use it consistently. For CS106A, we recommend just using single quote. Single quote is tiny bit easier since it does not require the shift key. Not thinking about which quote mark to use will free up valuable brain cells for something.
2 Blank Lines Before Def
PEP8 requires 2 blank lines before each def in a file. This is one of the weaker PEP8 rules. We will not be very upset about your code if it has 3 lines between functions.
Blank Lines Within a Def
If the lines of code have have natural phases — a few lines setting up the file, a few lines sorting the keys — use blank lines within the def to separate these phases from each other, much like dividing an essay into paragraphs. The standard says to use blank lines 'sparingly'.
Prefer !=
and not in
This is a preference between two equivalent forms which both work correctly. We prefer the 'shortcut' forms for not-equal and not-in testing, like this:
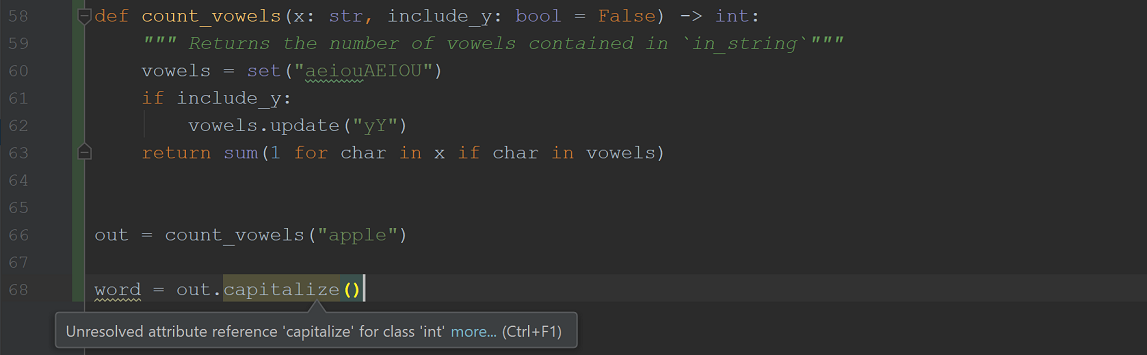
You can also make pep8.py show the error text in different formats by using –format having options default/pylint/custom:
Variables in the custom
format option
Variable | Significance |
---|---|
path | File name |
row | Row number |
col | Column number |
code | Error code |
text | Error text |
Quick help is available on the command line:
The behaviour may be configured at two levels, the user and project levels.
At the user level, settings are read from the following locations:
- If on Windows:
~.pep8
- Otherwise, if the
XDG_CONFIG_HOME
environment variable is defined: XDG_CONFIG_HOME/pep8
- Else if
XDG_CONFIG_HOME
is not defined: ~/.config/pep8
Example:
At the project level, a setup.cfg
file or a tox.ini
file is read ifpresent (.pep8
file is also supported, but it is deprecated). If none ofthese files have a [pep8]
section, no project specific configuration isloaded.
This is the current list of error and warning codes:
code | sample message |
---|---|
E1 | Indentation |
E101 | indentation contains mixed spaces and tabs |
E111 | indentation is not a multiple of four |
E112 | expected an indented block |
E113 | unexpected indentation |
E114 | indentation is not a multiple of four (comment) |
E115 | expected an indented block (comment) |
E116 | unexpected indentation (comment) |
E121 (*^) | continuation line under-indented for hanging indent |
E122 (^) | continuation line missing indentation or outdented |
E123 (*) | closing bracket does not match indentation of opening bracket's line |
E124 (^) | closing bracket does not match visual indentation |
E125 (^) | continuation line with same indent as next logical line |
E126 (*^) | continuation line over-indented for hanging indent |
E127 (^) | continuation line over-indented for visual indent |
E128 (^) | continuation line under-indented for visual indent |
E129 (^) | visually indented line with same indent as next logical line |
E131 (^) | continuation line unaligned for hanging indent |
E133 (*) | closing bracket is missing indentation |
E2 | Whitespace |
E201 | whitespace after ‘(‘ |
E202 | whitespace before ‘)' |
E203 | whitespace before ‘:' |
E211 | whitespace before ‘(‘ |
E221 | multiple spaces before operator |
E222 | multiple spaces after operator |
E223 | tab before operator |
E224 | tab after operator |
E225 | missing whitespace around operator |
E226 (*) | missing whitespace around arithmetic operator |
E227 | missing whitespace around bitwise or shift operator |
E228 | missing whitespace around modulo operator |
E231 | missing whitespace after ‘,', ‘;', or ‘:' |
E241 (*) | multiple spaces after ‘,' |
E242 (*) | tab after ‘,' |
E251 | unexpected spaces around keyword / parameter equals |
E261 | at least two spaces before inline comment |
E262 | inline comment should start with ‘# ‘ |
E265 | block comment should start with ‘# ‘ |
E266 | too many leading ‘#' for block comment |
E271 | multiple spaces after keyword |
E272 | multiple spaces before keyword |
E273 | tab after keyword |
E274 | tab before keyword |
E3 | Blank line |
E301 | expected 1 blank line, found 0 |
E302 | expected 2 blank lines, found 0 |
E303 | too many blank lines (3) |
E304 | blank lines found after function decorator |
E4 | Import |
E401 | multiple imports on one line |
E402 | module level import not at top of file |
E5 | Line length |
E501 (^) | line too long (82 > 79 characters) |
E502 | the backslash is redundant between brackets |
E7 | Statement |
E701 | multiple statements on one line (colon) |
E702 | multiple statements on one line (semicolon) |
E703 | statement ends with a semicolon |
E704 (*) | multiple statements on one line (def) |
E711 (^) | comparison to None should be ‘if cond is None:' |
E712 (^) | comparison to True should be ‘if cond is True:' or ‘if cond:' |
E713 | test for membership should be ‘not in' |
E714 | test for object identity should be ‘is not' |
E721 (^) | do not compare types, use ‘isinstance()' |
E731 | do not assign a lambda expression, use a def |
E9 | Runtime |
E901 | SyntaxError or IndentationError |
E902 | IOError |
W1 | Indentation warning |
W191 | indentation contains tabs |
W2 | Whitespace warning |
W291 | trailing whitespace |
W292 | no newline at end of file |
W293 | blank line contains whitespace |
W3 | Blank line warning |
W391 | blank line at end of file |
W5 | Line break warning |
W503 | line break occurred before a binary operator |
W6 | Deprecation warning |
W601 | .has_key() is deprecated, use ‘in' |
W602 | deprecated form of raising exception |
W603 | ‘<>' is deprecated, use ‘!=' |
W604 | backticks are deprecated, use ‘repr()' |
(*) In the default configuration, the checks E121, E123, E126,E133, E226, E241, E242 and E704 are ignored because theyare not rules unanimously accepted, and PEP 8 does not enforce them. Thecheck E133 is mutually exclusive with check E123. Use switch --hang-closing
to report E133 instead of E123.
(^) These checks can be disabled at the line level using the #noqa
special comment. This possibility should be reserved for special cases.
Note: most errors can be listed with such one-liner:
The flake8 checker is a wrapper aroundpep8
and similar tools. It supports plugins.
Other tools which use pep8
are referenced in the Wiki: list of relatedtools.
CS106A does not just teach coding. It has always taught how to write clean code with good style.Your section leader will talk with you about the correctness of code, but also pointers for good style.
Messy code works poorly — hard to read, hard to debug. To help you develop the right habits, we want you to always turn in clean code, and of course all of our examples should also have good clean style.
The goal of good style is 'readable' code, meaning that when someone sweeps their eye over the code, what that code does shines through.
Experience has shown that readable code is less time consuming to build and debug. Debugging especially can be a huge time-sink, so learning to write clean code and keep it clean is how we will do things all the time. Like surgeons keeping their hands clean, it's the right way to do it and we will try to develop the habit all the time.
Readability 1 - Function and Variable Names
The first step to good readability is a good variable and function names, like this example:
The narrative steps the code takes are apparent from the good function and variable names (essentially the verbs and nouns in the narrative). No other comments are necessary here. The names alone make it very readable.
The functions are the actions in the code, so we prefer verb names like 'display_alert' or 'remove_url' that say what the function does. Variables are like the nouns of the code, so we prefer names that reflect what that variable stores like 'urls' or 'original_image' or 'resized_image'. We'll talk about naming in more detail in the Style 2 document.
Readability 2 - PEP8 Tactics
Python is developed as a collaborative, free and open source project. A 'PEP' (Python Enhancement Proposal) is a written proposal used in Python development.
One of the earliest PEPs, PEP8, is a consensus set of style and formatting rules for writing 'standard' style Python, so your code has the right look for anyone else reading or modifying it. This is very much like English spelling reform, where 'public' is the standard spelling, and 'publick' is out of use and looks weird.
Here are the most important PEP8 rules we will use. The code you turn in should abide by PEP8.
Indent 4 Spaces
Indent code logically using spaces — 4 spaces is the most common.For if/for/while .. write 'body' lines indented on the next line after the colon.Early in the life of Python, some programs used 2 spaces and some used 4 spaces, but 4 spaces has grown to dominate. The best practice is to use literal spaces to indent the code, not tab stops.
Single Space Between Items
Most lines of code have a mix of variables and 'operators' such as as + * / = >=
. Use a single space to separate the operators and items:
Space After Comma/Colon
A comma or colon has 1 space after it, but no spaces before it.
Parenthesis No Space
The left and right parenthesis are not separated from what they contain, and the parenthesis for a function call is not separated from the name of the function. Similarly, square brackets and curly braces are not separated from what they contain.
Use a Consistent Quote Mark
Python strings can be written within single quotes like 'Hello'
or double quotes like'there'
. PEP8 requires a program to pick one quote style and use it consistently. For CS106A, we recommend just using single quote. Single quote is tiny bit easier since it does not require the shift key. Not thinking about which quote mark to use will free up valuable brain cells for something.
2 Blank Lines Before Def
PEP8 requires 2 blank lines before each def in a file. This is one of the weaker PEP8 rules. We will not be very upset about your code if it has 3 lines between functions.
Blank Lines Within a Def
If the lines of code have have natural phases — a few lines setting up the file, a few lines sorting the keys — use blank lines within the def to separate these phases from each other, much like dividing an essay into paragraphs. The standard says to use blank lines 'sparingly'.
Prefer !=
and not in
This is a preference between two equivalent forms which both work correctly. We prefer the 'shortcut' forms for not-equal and not-in testing, like this:
These forms arrange the code more in line with the natural phrasing, like 's is not donut' or 'donut is not in foods'.
Do Not Write: if x True
Pep8 Args
The way that boolean True/False is evaluated in an if/while test is a little more complicated than it appears. The simple rule is this: do not write if x True
or if x False
in an if/while test.
Say we have a print_greeting()
function that takes in a shout_mode
parameter.
Do not write this:
The if
structure can distinguish True vs. False values itself. Therefore, write the code to give the boolean shout_mode
to the if
directly, like this:
To invert the logic do not use False
. Use not
like this:
Remove Pass from Code
The pass
directive in Python is a placeholder line that literally does nothing. It is very rarely used in regular production code. However, in coding exercises, there is often some boilerplate code with a pass
marking the spot for the student code. Remove all the pass
markers from your code before turning it in.
# Inline Comments
It's a good practice to add # 'inline' comments to explainwhat especially non-obvious or interesting code does.Inline comments are not needed when the code itself does a goodjob of showing what the line does.
1. For the following lines, the code does a good job of tellingthe story, so no inline comments are needed beyond the good variableand function names. Most lines of code are like this.
In particular, avoid putting in a comment that restates what the code itself says.
2. Inline comments are appropriate as in theexample below, explainingwhat a non-obvious bit of code accomplishes -addressing the goal at work, not the re-stating the operators used.
See how theinline comment provides useful informationthat is not obvious from the line itself.
3. Another important use of inline commentsto note when the code does not do what itappears to. This speaks to readability -the code does not do what its variable and functionnames suggest, so an inline commentcan help clear things up.
Example code used in teaching often has more inline comments than regular production code, since for teaching there are many times we want to point out or explain a technique shown on a line.
Named Parameter Exception
There is an exception to the 1-space-between-items rule, but it's for a rare case. If a function call has named parameters, no spaces are needed around the =
like this:
I think the reasoning here is that this use of =
is different the more common variable assignment =
, so it's good to make this use look a little different.
The Misguided PEP8 Rule About
How do you compare two values in Python? The answer is: use the operatorlike this:
It works for strings, it works for ints, it works for lists, it works for pretty much everything. Having nailed down that is the simple, correct thing that should come to mind when comparing two values, you may skip the following paragraph and simply use with an uncluttered mind.
There is a IMHO misguided requirement in PEP8: it requires that comparisons to the special value None use the obscure is
operator instead of . That is a bad idea, since comparing to None is pretty common, but the is
operator is quite obscure. In fact it's unusual for a program to have any need for the is
operator at all.Forcing the mass of regular programs to use this boutique operator most people have never heard of and will never need is not good Python. Therefore, the CS106A position it to just use to compare any two values, which is the simple, intuitive way to think about comparisons.
Naming quirks:
Python Keywords
A few words like def
, for
, and if
are fixed 'keywords' in Python, so you cannot use those words as variable or function names. Here is the list of keywords:
The 'len' Rule: Do Not Use Builtin Functions as a Variable Names
Python has many built-in functions like len()
and min()
. As a strange example of Python's flexibility, your code can use assignment =
to change what len
means within your code .. probably a huge mistake!
Here is the simple rule: do not use the name of a common function as a variable name. Here are the most common function names:
Pep8 Download
Rather than memorize the list of all the function names to avoid, it is sufficient to avoid using the names of functions that your code uses, typically the well known functions like len
, range
, min
, and so on.
Incidentally, this is why we avoid using 'str' or 'list' as variable names, instead using 's' and 'lst'.
Redefining a function in this way only affects your local code, not code elsewhere in the program. So if your code accidentally defines a variable named divmod
, that will not interfere with code in another function calling divmod()
.
A Little Readability Joke
Pep 8 Comments
This is an optional point for anyone who reads this far down. What does readability mean? One could say it means the visuals of the code fit with the semantics of what it's going to do.
With that in mind, what do you think about this code that computes a total score, but the spacing technically violates PEP8:
I'd say it looks ok. The spaces are missing, but the missing spaces are in agreement with the precedence — the multiplication will happen first, and the missing spaces sort of reinforce that.
What about this version?
You may have chuckled or winced a little looking at this one. It is just so wrong, sort of like the punchline of a joke. This may give you a little insight that the meaning of code and its appearance have some deep linkage. Given that linkage, it's maybe not surprising that messy looking code is more prone to confusion and bugs.
Links For SLs
Here are links to the sections for particular style rules, handy for pasting into grading notes.
Copyright 2020 Nick Parlante